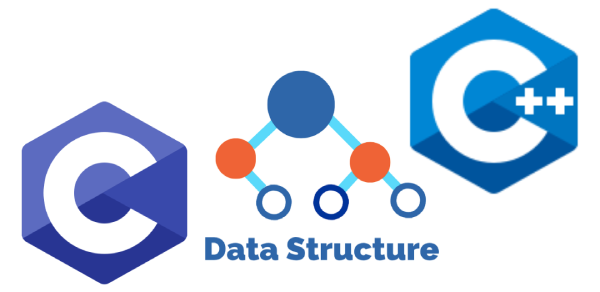
Foundation of Programming
Programming Foundations
Understanding the C programming language is essential as it forms the basic syntax and structure of most modern languages.
The use of C++ with STL introduces object-oriented concepts and standard libraries, which are pivotal for complex problem solving.
Grasping data structures is crucial for managing data efficiently, which is the backbone of algorithm development and computational thinking.
These foundational skills are a prerequisite for tackling advanced topics like web development, database management, and application development, ensuring a well-rounded skill set for any aspiring programmer.
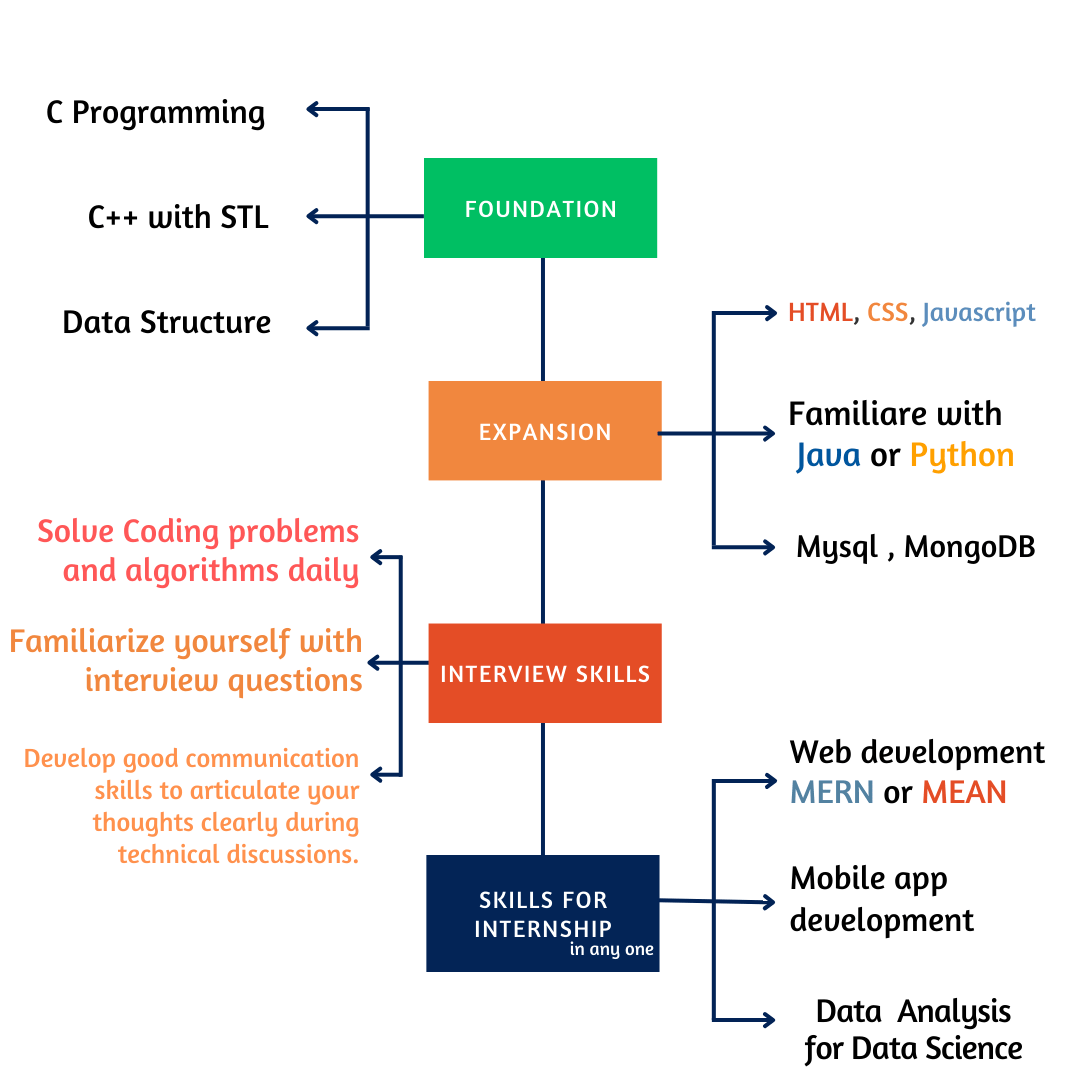
C Programming
- Introduction to C programming
- Basic data types and operations
- Control flow and loops
- Functions
- Arrays and pointers
- Structures and unions
- File input/output
- Advanced topics
- Standard Library Functions
- Debugging and error handling
- Project Work
C plus plus
- Introduction to C++
- Basic Syntax
- Object-Oriented Programming
- Templates and Generic Programming:
- Memory Management
- Exception Handling
- File Input/Output
- Standard Template Library (STL)
- Advanced Topics
- Debugging and testing
Data Structure
- Brush up C Language Data Types, Constants & Variables
- Structure
- Linked list
- Stack
- Queue
- Liner Search, Binary Search
- Sorting
- Tree
- Graph